How to Center a Div in CSS ? Let’s explore some straightforward techniques for centering a div without wasting much time.
1. Flexbox Magic:
Flexbox is a layout model in CSS that makes it easy to design complex layouts. In this method, the parent container becomes a flex container, and the align-items: center
and justify-content: center
properties ensure both horizontal and vertical centering.
.container { display: flex; align-items: center; justify-content: center; height: 100vh; } div { width: 50%; height: 50%; }
2. Grid Goodness:
CSS Grid is a two-dimensional layout system. The parent container is set as a grid container, and the place-items: center
property centers the div both horizontally and vertically.
.container { display: grid; place-items: center; height: 100vh; } div { width: 50%; height: 50%; }
3. Absolute Simplicity:
This method utilizes absolute positioning and the transform
property to center the div. The parent container is set to position: relative
, and the child div is absolutely positioned at 50% from the top and left, then translated back by 50% of its own size.
.container { position: relative; height: 100vh; } div { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); width: 50%; height: 50%; }
4. Table-cell Trickery:
By mimicking the behavior of a table, this method utilizes display: table
for the container and display: table-cell
for the div. The vertical-align: middle
ensures vertical centering, and text-align: center
handles horizontal centering.
.container { display: table; height: 100vh; width: 100%; } div { display: table-cell; vertical-align: middle; text-align: center; width: 50%; height: 50%; }
5. Line-height Charm:
Leveraging the line-height
property, this method centers the div vertically by setting the line height of the container to the full viewport height. The div, set as an inline-block, aligns vertically in the middle.
.container { height: 100vh; width: 100%; text-align: center; } div { display: inline-block; line-height: 100vh; vertical-align: middle; width: 50%; height: 50%; }
Conclusion:
To sum it up, there are different ways to center a div both horizontally and vertically in CSS. You can use properties like display: flex and display: grid, or go for position: absolute with transform: translate. Another option is to use display: table and display: table-cell, or simply adjust the line-height.
Each method has its own pros and cons, and the best choice depends on your project’s needs. Regardless of the method, centering a div in CSS can make your webpage look better and be more user-friendly.
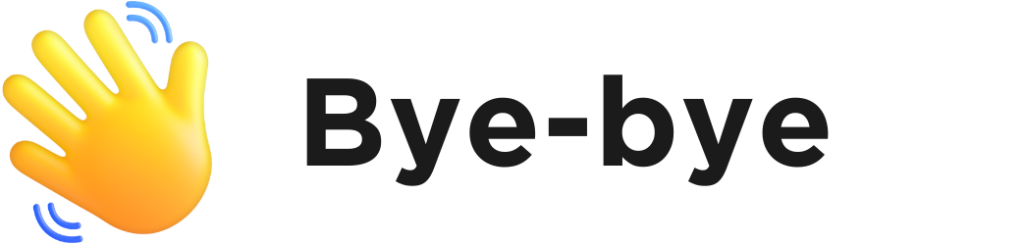