Table of Contents
Start mastering JavaScript with ‘JavaScript Roadmap: From Fundamentals to Expert Level.’ Follow along from basics to advanced skills with clear guidance and practical exercises, empowering you to become a JavaScript pro.
References
- Cheat Sheet : Link
- All JavaScript Details : Javascript.info
- JavaScript Documentation : MDN Docs
- Books : Eloquent JS | You Don’t Know JS
Basics
- Console logging
- Variable & Datatypes (using var)
- Number: Used for numbers, both whole (integers) and with decimal points (floating point numbers).
- Strings: Used for text data.
- Boolean: Represents true or false values.
- Undefined: Type of a variable that’s declared but not assigned a value.
- Null: Represents the intentional absence of any value.
- Dynamic Typing: JavaScript automatically assigns data types.
- Camel Case and Naming Conventions: Guidelines for naming variables and functions in JavaScript.
- Comments
- Mutation (Changing Data in variable) & Coercion (Data type conversion)
- Math Operators & Logic Operators
+ , - , * , / , %
- < , > and <= , >= …
- Compare letters according to Unicode (‘a’ < ‘b’)
typeof
& (== & ===)- Operator Precedence & Multiple Assignments
- Operators Shorthand
- Conditionals : If else, nested If, if else if
- Ternary operator
- Switch Statement
- Boolean Logic : && , || , !
- Truthy and Falsy values
- Falsy values : undefined , null , 0 , ”, NaN
- Truthy values : all others give true on coercion
- Loops & Iteration
- For Loop
- While Loop
- Continue & Break
- Error Handling
- Throw
- try , catch
- Strict Mode (
"use strict"
)- Strict Mode (“use strict”) catches common coding errors by throwing exceptions.
- It prevents or throws errors when potentially “unsafe” actions occur, like accessing the global object.
- Strict Mode disables confusing or poorly thought-out features.
Functions
- Basic Functions ( declaration , returning data )
- Function Statement (Direct Declaration):
- You declare a function directly like
function myFunction() { ... }
. - When you use it, it gives you nothing (
undefined
) in certain situations like insideif
orwhile
statements.
- You declare a function directly like
- Function Expression (Using Variable):
- You assign a function to a variable like
const myFunction = function() { ... }
. - This always gives you something back, whether it’s the type of the function, the variable itself, or a result like
2 + 3
.
- You assign a function to a variable like
- Function Statement (Direct Declaration):
- Default Parameters
- Callback Functions – Functions as Arguments & Return Functions from Functions
- IIFE – Immediately Invoked Function Expression
- It’s like making a secret little function inside braces
{}
and then using it right away. - You can only access what this function gives back, which helps keep things private and organized, sort of like hiding secrets.
- It’s like making a secret little function inside braces
- Closures
- Inner functions can still use variables and parameters from their outer function, even after the outer function is done running.
- This is because of the Scope Chain, which is like a pointer. Even when the outer function is finished, the Scope Chain still keeps track of its variables, so inner functions can still access them.
- Argument Object
- Inbuilt String methods (indexOf , startsWith, substring)
- Inbuilt Number Methods (MATH object)
- Other Useful Inbuilt Functions :
- Date function
- Split & Join function
- Set timeout
- ParseInt
Arrays
- Basic Arrays (declaration)
New Array ()
Syntax- Array Properties : length , index
- Array Methods : pop , push , shift , unshift
- Iterating Arrays :
for...of
,for...in
, for each, map - Searching Arrays :
indexOf , find , findIndex
- Filtering arrays : filter , reduce
- Sorting Arrays : sort
- Altering Arrays : split and join functions
- Useful Array Functions : splice , slice , concat , reverse , every , some
Objects & Properties
- Basic Objects (declaration , accessing , mutating)
- new Object () Syntax
- Functions in objects (Are methods)
- Prototypes & Prototype chains
- Prototypes serve as inheritable methods within objects.
- Inherited objects inherently access their parent’s prototype.
- Methods designated for inheritance are specifically placed within the prototype.
- The prototype of a constructor extends to all of its instances.
- Object prototypes can be inspected via
object.property
orobject.__proto__
. - The
hasOwnProperty
method discerns whether a property is inherited. instanceOf
is utilized to determine an object’s specific instance within its inheritance chain.
- Constructors – used as a blueprint to create multiple objects
- Constructor Functions – used to initialize data of object for every instance
- Constructors are formed through functions.
- The convention dictates starting constructor names with a capital letter.
- Object variables are initialized using the
this
keyword. - Instances are generated with the
new
keyword followed by the constructor name. - Prototypes are established via
object.prototype
. - Inheritance from other constructors is achieved using the
call
method with parent constructor parameters. - Constructors can alternatively be crafted using
Object.create
, specifying the prototype before the data. - Inheriting a parent’s prototype for a child prototype is done through
Object.create(parent.prototype)
.
- Primitives & Object
- Primitives directly contain data (e.g., numbers, strings).
- Objects reference other objects (e.g., objects, arrays).
- In JavaScript, nearly everything is an object.
- Primitives include numbers, strings, booleans, undefined, and null.
- Primitives can be automatically converted to objects (autoboxing) when certain methods, like
string.length
, are invoked. - Everything else, such as arrays, functions, and objects themselves, are objects.
- Two objects are only equal if they take the same space and position in memory , they wont be equal no matter the keys and properties.
- Bind, Call & Apply
- Call: Changes the value of
this
and immediately invokes the function. - Apply: Similar to call, but arguments are passed as an array.
- Bind: Sets the value of
this
and other arguments for a function, creating a new function that can be called later with those preset values.
- Call: Changes the value of
Document Object Model (DOM)
- DOM
- structured representation of HTML
- DOM connects webpages to scripts like JS
- for each HTML box there is an object in DOM that we can access and interact with
- DOM Methods – querySelecor, getElementById
- Event Listeners – storage , UI listener (mouse)
- Data Storage CRUD – local Storage
window.location
– assign , hash- window – inner width , inner height, console, document, addeventlistner(to work on multiple tabs)
ES6+
- let and const
let
andconst
are only block-scoped, meaning they’re only accessible within the block they’re declared in (likeif
orwhile
blocks).var
, on the other hand, is function-scoped.- Variables declared with
let
andconst
cannot be used before they’re declared, whilevar
variables are initialized with a value ofundefined
when accessed before declaration. - Immediately Invoked Function Expressions (IIFE) can be created in ES6 using block scopes with
{}
, allowing for immediate execution of a function within a block.
- Template Strings
- Arrow Functions (lexical this keyword functionality)
- Destructuring :
{ name , length } = object
,[name , length] = array
- Spread Operator : spread array , objects
- Rest Parameters
- Maps : same like object , but keys can be numbers, functions anything
- we can loop through them
- functions : get,set, size , has, delete, clear, entries
- Class : syntactical sugar for es5 constructor and inheritance
- Class Constructor
- Class methods
- Subclasses , Super & Extends
- Getters and Setters
Asynchronous JavaScript
- Asynchronous Functions can run in background
- HTTP requests & response (Old Way)
XMLhttprequest
,readystatechange
- readyState, open, send
- Promises
- A promise keeps track of something that’s supposed to happen and what should be done afterwards.
- It represents something we expect to get in the future.
- Promises can be in one of four states: waiting to happen, done successfully, completed, or failed.
- We use
resolve
andreject
to check and handle what happens with the promise. - We handle successful outcomes with
.then
and errors with.catch
. - Promises let us link several things we need to do, one after the other, even if they’re happening at the same time.
- Async/Await alternative way to consume promises
- we can use the response promise given by await to check and catch errors.
- Fetch API: It provides promises that we can handle using
async/await
or regular promises. - Axios: This is an alternative to Fetch. It directly gives us data in a JSON format.
- API: Stands for Application Programming Interface. It’s like a remote server’s special area that lets us interact with it.
- JSON: Stands for JavaScript Object Notation. It’s a format to represent data in JavaScript. JavaScript has a built-in
json
function to handle it. - AJAX: Stands for Asynchronous JavaScript and XML. It’s a technique to send and receive data from a server without reloading the webpage.
- CrossOrigins
Conclusion
In conclusion, mastering JavaScript from fundamentals to expert level opens up a world of possibilities in web development.
This marks just the beginning of your journey in JavaScript! There’s always so much more to explore and learn. Keep pushing yourself, experimenting with new concepts, and diving deeper into the world of JavaScript. The possibilities are endless, and every new discovery brings you closer to mastering this powerful language.
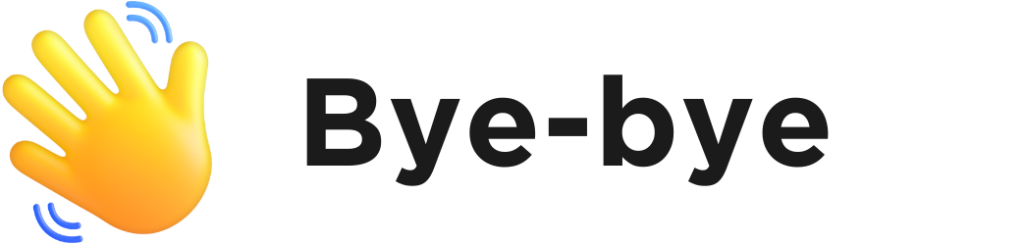