Discover instant enhancements for your web projects with ’20 JavaScript Tips and Tricks You Can Use Right Now.’ Elevate your coding skills with practical insights and unleash the full potential of JavaScript in your development endeavors.
JavaScript, the language that makes websites do cool stuff, has some awesome tricks that can make your coding easier and more fun. In this post, we’ll check out 20 simple and cool tips for JavaScript, each explained with easy examples. Let’s jump in and make your JavaScript skills even better! ๐
1. Unwrapping Goodness: Effortless Value Extraction
Destructuring simplifies unpacking values from arrays or objects. Witness the magic:
const car = { brand: 'Tesla', model: 'Model 3' }; const { brand, model } = car; console.log(brand); // Result: Tesla console.log(model); // Result: Model 3
2. Share the Joy: Clone Arrays and Merge Objects
The spread operator (...
) effortlessly duplicates arrays and merges objects:
const originalArray = [4, 5, 6]; const copiedArray = [...originalArray]; console.log(copiedArray); // Result: [4, 5, 6]
Merging objects:
const obj1 = { x: 10, y: 20 }; const obj2 = { y: 30, z: 40 }; const mergedObj = { ...obj1, ...obj2 }; console.log(mergedObj); // Result: { x: 10, y: 30, z: 40 }
3. The Might of map()
: Transforming with Grace
The map()
method is your ally for effortless data transformations:
const prices = [25, 30, 35]; const discountedPrices = prices.map(price => price * 0.9); console.log(discountedPrices); // Result: [22.5, 27, 31.5]
4. Swift Moves with &&
and ||
: Elegant Conditionals
Employ &&
and ||
for neat and concise conditionals:
const username = userProfile.username || 'Guest'; console.log(username); // Result: Guest
5. Sequencing Delays with Chained setTimeout()
Chaining setTimeout()
orchestrates a series of delayed actions:
function delayedGreet(message, time) { setTimeout(() => { console.log(message); }, time); } delayedGreet('Greetings!', 1500); // Result (after 1.5 seconds): Greetings!
6. Arrow Functions: Short and Potent
Arrow functions (() => {}
) are not just concise; they also preserve the value of this
:
const shout = message => `Attention! ${message}`; console.log(shout('Important message!')); // Result: Attention! Important message!
7. Proficiency in Promise.all()
: Managing Multiple Promises
Merge and handle multiple promises using Promise.all()
:
const promiseA = fetch('urlA'); const promiseB = fetch('urlB'); Promise.all([promiseA, promiseB]) .then(responses => console.log(responses)) .catch(error => console.error(error));
8. Dynamic Property Names: Adaptable Object Keys
Utilize variables as object property names using square brackets:
const attribute = 'color'; const carDetails = { [attribute]: 'blue' }; console.log(carDetails.color); // Result: blue
9. Magical Template Literals: String Formatting
Template literals (${}
) enable you to embed expressions in strings:
const itemName = 'Laptop'; const message = `You've added ${itemName} to your cart.`; console.log(message); // Result: You've added Laptop to your cart.
10. NaN Safeguard: A Secure Alternative
Use Number.isNaN()
for precise NaN checks:
const notANumber = 'Invalid input'; console.log(Number.isNaN(notANumber)); // Result: false
11. Optional Chaining (?.
): Tame Undefined Values
Avoid errors with optional chaining when dealing with nested properties:
const user = { info: { name: 'Stella' } }; console.log(user.info?.age); // Output: undefined
12. Regex Revival: Mastering Patterns
Regular expressions (RegExp
) are powerful tools for pattern matching:
const text = 'Hello, Stella!'; const pattern = /Hello/g; console.log(text.match(pattern)); // Output: ['Hello']
13. JSON.parse() Reviver: Transform Parsed Data
The reviver
parameter in JSON.parse()
lets you transform parsed JSON:
const data = '{"age":"20"}'; const parsed = JSON.parse(data, (key, value) => { if (key === 'age') return Number(value); return value; }); console.log(parsed.age); // Output: 20
14. Cool Console Tricks: Debugging Delights
Go beyond console.log()
with console.table()
and console.groupCollapsed()
:
const newUsers = [{ name: 'Eve' }, { name: 'Charlie' }]; console.table(newUsers); console.groupCollapsed('Details'); console.log('Name: Eve'); console.log('Age: 25'); console.groupEnd();
15. Fetch with async
/await
: Asynchronous Simplicity
async
/await
with fetch()
simplifies handling asynchronous requests:
async function fetchData() { try { const response = await fetch('url'); const data = await response.json(); console.log(data); } catch (error) { console.error(error); } } fetchData();
16. Closures Unleashed: Data Privacy
Closures let you create private variables in functions:
function createCounter() { let count = 0; return function () { count++; console.log(count); }; } const counter = createCounter(); counter(); // Output: 1 counter(); // Output: 2
17. Memoization for Speed: Efficient Recalculation
Memoization caches function results for improved performance:
function fibonacci(n, memo = {}) { if (n in memo) return memo[n]; if (n <= 2) return 1; memo[n] = fibonacci(n - 1, memo) + fibonacci(n - 2, memo); return memo[n]; } console.log(fibonacci(10)); // Output: 55
18. Hail the Intersection Observer: Effortless Scroll Effects
Use the Intersection Observer API for lazy loading and scroll animations:
const observer = new IntersectionObserver(entries => { entries.forEach(entry => { if (entry.isIntersecting) { entry.target.classList.add('fade-in'); observer.unobserve(entry.target); } }); }); const elements = document.querySelectorAll('.animate'); elements.forEach(element => observer.observe(element));
19. ES6 Modules for Clean Code: Organized and Modular
Use ES6 modules for clean, modular code:
// math.js export function add(a, b) { return a + b; } // app.js import { add } from './math.js'; console.log(add(3, 7)); // Output: 10
20. Proxies: Beyond Objects
Proxies allow you to intercept and customize object operations:
const handler = { get(target, prop) { return `Property "${prop}" doesn't exist.`; } }; const proxy = new Proxy({}, handler); console.log(proxy.name); // Output: Property "name" doesnโt exist.
Conclusion
With these 20 JavaScript tips and tricks, you’ve got a powerful toolkit to elevate your coding skills. ๐ Keep exploring, experimenting, and building awesome things with JavaScript! Remember, the journey of coding is as exciting as the destination.
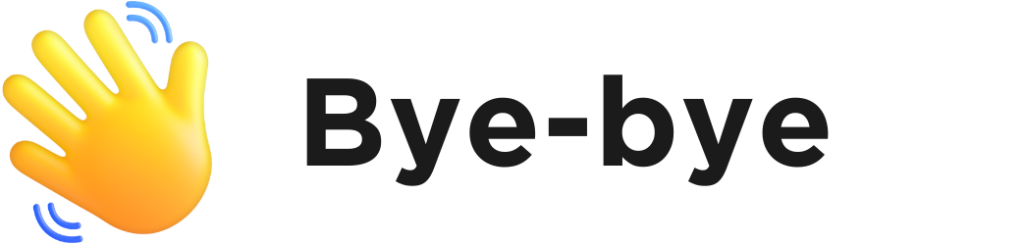