Discover 15 advanced web development techniques that don’t rely on JavaScript. From responsive design to efficient CSS animations, explore innovative approaches to building dynamic and interactive websites without traditional scripting languages.
We’ll show you real examples and compare them to older ways that used JavaScript, to demonstrate how powerful modern web tech can be.

1. Responsive Typography
In the past, JavaScript was commonly used to adjust font sizes according to screen sizes. However, with the introduction of CSS Custom Properties (Variables) and the clamp()
function, creating responsive typography has become much simpler.
Old Approach (JavaScript):
<p id="responsiveText">This is some text</p> <script> const screenWidth = window.innerWidth; const baseFontSize = (screenWidth > 768) ? '20px' : '16px'; document.getElementById('responsiveText').style.fontSize = baseFontSize; </script>
New Approach (CSS Custom Properties):
:root { --base-font-size: 16px; } p { font-size: clamp(var(--base-font-size), 5vw, var(--base-font-size * 1.25)); }
CSS Custom Properties (Variables) and the clamp()
function enable the creation of a responsive and scalable typography system entirely using CSS, eliminating the need for JavaScript.
2. Dark Mode Switch
The prefers-color-scheme
media query combined with CSS Custom Properties enables the implementation of a dark mode switch without JavaScript, providing a seamless dark mode experience solely through CSS styling.
Old Approach (JavaScript):
<label class="switch"> <input type="checkbox" onclick="toggleDarkMode()"> <span class="slider"></span> </label> <script> function toggleDarkMode() { document.body.classList.toggle('dark-mode'); } </script>
New Approach (CSS and Media Query):
:root { --background-color: #fff; --text-color: #000; } @media (prefers-color-scheme: dark) { :root { --background-color: #333; --text-color: #fff; } } body { background-color: var(--background-color); color: var(--text-color); }
Dark mode can be achieved seamlessly using only CSS custom properties and the prefers-color-scheme
media query, eliminating the need for JavaScript.
3. Interactive Hover Transitions
Intricate hover transitions that previously required JavaScript can now be accomplished using only the transition
property and advanced CSS pseudo-elements, completely eliminating the need for JavaScript.
Old Approach (JavaScript):
<div onmouseover="expandElement(this)" onmouseout="shrinkElement(this)">Hover me</div> <script> function expandElement(element) { element.style.transform = 'scale(1.2)'; } function shrinkElement(element) { element.style.transform = 'scale(1)'; } </script>
New Approach (CSS):
div { transition: transform 0.3s ease-in-out; } div:hover { transform: scale(1.2); }
The transition
property streamlines hover effects, providing smooth and interactive transitions without relying on JavaScript.
4. Placeholder Animations
Stylish and dynamic placeholder animations in input fields can now be achieved using the ::placeholder
pseudo-element in CSS, eliminating the need for JavaScript.
Old Approach (JavaScript):
<input type="text" onfocus="animatePlaceholder(this)" onblur="resetPlaceholder(this)"> <script> function animatePlaceholder(input) { input.placeholder = 'Type something...'; } function resetPlaceholder(input) { input.placeholder = ''; } </script>
New Approach (CSS):
input::placeholder { transition: all 0.3s ease-in-out; } input:focus::placeholder { transform: translateY(-100%); opacity: 0.7; }
CSS animations applied to the ::placeholder
pseudo-element create visually appealing effects when an input field is focused, enhancing the user experience without relying on JavaScript.
5. Image Lazy Loading
The loading
attribute in the img
element provides a native solution for lazy loading images, eliminating the need for JavaScript in this common task.
Old Approach (JavaScript):
<img data-src="image.jpg" alt="Lazy-loaded Image" id="lazyImage"> <script> document.addEventListener('DOMContentLoaded', function () { const lazyImage = document.getElementById('lazyImage'); lazyImage.src = lazyImage.dataset.src; }); </script>
New Approach (HTML Loading Attribute):
<img src="image.jpg" alt="Lazy-loaded Image" loading="lazy">
The loading="lazy"
attribute ensures that images are lazily loaded by the browser, reducing reliance on custom JavaScript implementations for lazy loading functionality.
6. Scroll-Triggered Animations
The scroll-margin-top
CSS property enables triggering animations based on scroll position without the need for JavaScript.
Old Approach (JavaScript):
<div class="animate-me" onscroll="animateElement(this)">Scroll to animate</div> <script> function animateElement(element) { // JavaScript animation logic // ... } </script>
New Approach (CSS):
.animate-me { opacity: 0; transition: opacity 0.5s; scroll-margin-top: 20vh; } .animate-me.in-view { opacity: 1; }
CSS, along with the scroll-margin-top
property, enables smooth scroll-triggered animations without relying on JavaScript.
7. Customizable Form Controls
The :focus-within
pseudo-class and CSS Custom Properties eliminate the need for JavaScript when styling form controls to match a specific design.
Old Approach (JavaScript):
<input type="text" class="custom-input" onfocus="highlightInput(this)" onblur="resetInput(this)"> <script> function highlightInput(input) { input.classList.add('focused'); } function resetInput(input) { input.classList.remove('focused'); } </script>
New Approach (CSS):
.custom-input { border: 2px solid var(--input-border); } .custom-input:focus-within { border: 2px solid var(--input-border-focused); } :root { --input-border: #ccc; --input-border-focused: #007bff; }
The :focus-within
pseudo-class and CSS Custom Properties offer a JavaScript-free approach to styling form controls with customizability.
8. Full-Page Overlay Menus
The :checked
pseudo-class, along with CSS, enables the creation of full-page overlays without the need for JavaScript.
Old Approach (JavaScript):
<input type="checkbox" id="menuToggle" onclick="toggleMenu()"> <label for="menuToggle">Open Menu</label> <script> function toggleMenu() { // JavaScript logic to toggle menu visibility // ... } </script>
New Approach (CSS and :checked):
#menuToggle { display: none; } #menuToggle:checked + label { /* Styles for open menu */ } label { cursor: pointer; }
The :checked
pseudo-class allows us to create full-page overlay menus without the need for JavaScript.
9. Gradient Borders
The conic-gradient
property in CSS lets us create gradient borders without needing complex CSS or JavaScript. It’s a simple way to achieve gradient borders directly in our stylesheets.
Old Approach (JavaScript):
<div class="gradient-border" onclick="toggleGradient()">Click to toggle gradient border</div> <script> function toggleGradient() { // JavaScript logic to toggle gradient border // ... } </script>
New Approach (CSS conic-gradient):
.gradient-border { border: 5px solid; border-image: conic-gradient(from 0deg at 50% 50%, red, yellow, green, blue, purple); border-image-slice: 1; }
The conic-gradient
property in CSS simplifies the creation of gradient borders, eliminating the need for JavaScript.
10. Multi-column Layouts
The column
property in CSS simplifies the creation of multi-column layouts, eliminating the need for JavaScript to dynamically adjust the layout.
Old Approach (JavaScript):
<div class="multi-column" onclick="adjustColumns()">Click to adjust columns</div> <script> function adjustColumns() { // JavaScript logic to adjust column layout // ... } </script>
New Approach (CSS column):
.multi-column { column-count: 3; column-gap: 20px; }
The column
property in CSS enables the creation of elegant multi-column layouts without the need for JavaScript.
11. Custom Checkbox and Radio Button Styles
With the :checked
pseudo-class and CSS, we can achieve custom styles for checkboxes and radio buttons without the need for JavaScript, aligning them with specific designs.
Old Approach (JavaScript):
<input type="checkbox" id="customCheckbox" onclick="toggleCheckbox()"> <label for="customCheckbox" class="custom-checkbox"></label> <script> function toggleCheckbox() { // JavaScript logic to toggle checkbox state // ... } </script>
New Approach (CSS and :checked):
input[type="checkbox"] { display: none; } input[type="checkbox"] + label { width: 20px; height: 20px; background-color: #ccc; display: inline-block; } input[type="checkbox"]:checked + label { background-color: #007bff; }
Using the :checked
pseudo-class, we can customize checkbox styles without relying on JavaScript.
12. Seamless Page Transitions
With the scroll-behavior
CSS property, smooth page transitions can be achieved without the need for JavaScript.
Old Approach (JavaScript):
<a href="#about" onclick="scrollToSection('about')">About</a> <script> function scrollToSection(section) { // JavaScript logic to scroll to the specified section // ... } </script>
New Approach (CSS scroll-behavior):
body { scroll-behavior: smooth; }
The scroll-behavior
property enables smooth scrolling without JavaScript event listeners.
13. Flexible Box (Flexbox) for Equal Height Columns
With Flexbox in CSS, equal height columns can be achieved effortlessly without the need for JavaScript.
Old Approach (JavaScript):
<div class="flex-container" onclick="adjustColumnHeights()">Click to adjust column heights</div> <script> function adjustColumnHeights() { // JavaScript logic to equalize column heights // ... } </script>
New Approach (CSS Flexbox):
.flex-container { display: flex; } .flex-container > div { flex: 1; }
The Flexbox layout in CSS simplifies the creation of flexible and equal-height columns, eliminating the need for JavaScript adjustments.
14. Dynamic Gradient Text
With CSS, we can now achieve dynamic gradient text effects easily, eliminating the need for complex JavaScript or SVG solutions.
Old Approach (JavaScript):
<div class="gradient-text" onclick="toggleGradientText()">Click to toggle gradient text</div> <script> function toggleGradientText() { // JavaScript logic to toggle gradient text // ... } </script>
New Approach (CSS linear-gradient):
.gradient-text { background-clip: text; color: transparent; background-image: linear-gradient(to right, #ff8c00, #ffcd00); }
Dynamic gradient text effects can be achieved using pure CSS with the background-clip property and linear-gradient.
15. Overlay Text on Images
Text overlay on images can be achieved easily using CSS with the position property, eliminating the need for JavaScript for positioning.
Old Approach (JavaScript):
<div class="image-container"> <img src="image.jpg" alt="Overlay Image"> <div class="overlay-text" onclick="toggleOverlayText()">Click to toggle overlay text</div> </div> <script> function toggleOverlayText() { // JavaScript logic to toggle text overlay position // ... } </script>
New Approach (CSS position):
.image-container { position: relative; } .overlay-text { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); }
The position
property simplifies the process of overlaying text on images, eliminating the need for JavaScript calculations.
Conclusion
Join us on an exciting adventure as we discover 15 advanced web development tricks that don’t use JavaScript. Learn how to make pages change smoothly, style checkboxes nicely, and make text look good on any device, all with just HTML and CSS.
Topics Covered:
- Responsive Typography: Use CSS Custom Properties and
clamp()
to make text responsive without JavaScript. - Dark Mode Switch: Implement dark mode using
prefers-color-scheme
and CSS custom properties, no JavaScript needed. - Interactive Hover Effects: Create hover transitions with CSS
transition
and pseudo-elements, avoiding JavaScript. - Dynamic Gradient Text: Achieve gradient text effects using CSS properties like
background-clip
andlinear-gradient
, no JavaScript required. - Equal Height Columns: Use CSS Flexbox for equal height columns without relying on JavaScript.
Start an exciting journey into web development, where HTML and CSS play key roles! We’ll reveal some neat tricks that enhance websites without using JavaScript.
As we wrap up, you’ll see the impressive power of HTML and CSS. These tricks make websites better without needing complex scripts.
From making text look good on any device to adding a button for light and dark modes, we’ll cover lots of fun things. These tricks showcase what modern web technology can achieve. With them, you can create cool websites that work smoothly and look fantastic, all while keeping your code clean.
Remember, it’s crucial to keep learning about HTML and CSS. As web development evolves, knowing these tricks helps you build websites that work well for everyone, without requiring lots of complicated code.
If you found these JavaScript-free techniques intriguing, be sure to follow @coding.stella for more insightful content. Stay tuned for in-depth tutorials, the latest trends, and practical insights that will keep you at the forefront of modern web development.
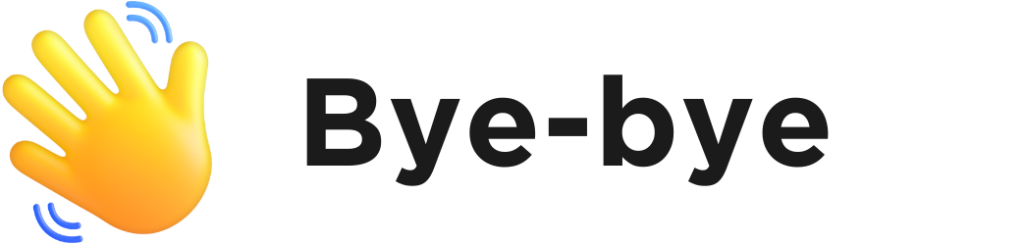